Validating Function Arguments in JavaScript: The Smart Way
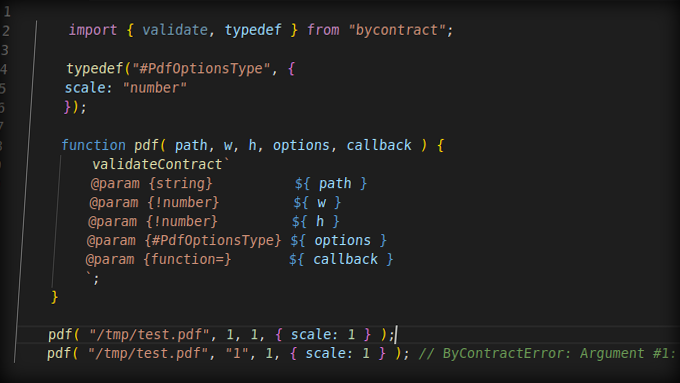
In software engineering we try to discover and eliminate bugs as soon as possible. One of most important heuristics here is validation of input/output on functions and methods. If you are going with TypeScript or Flow, you are fine. But if not? Then we have to manually validate at least input (arguments). But what would be the best way doing it?
First comes to mind aproba library. It’s “ridiculously” light-weight and equally popular:
import validate from "aproba";
function click( selector, x, y ) {
validate( "SNN", arguments );
}
Simple, isn’t it? For constraints we give a string where each character stands for a corresponding argument. So here we require selector to be a string (“S”) and both coordinates to be numbers (“N”).
But when it comes to optional arguments it is getting quite obscure. For example Node.js function fs.createWriteStream(path[, options]) maintainers of aproba recommend to describe as SO|SS|OO|OS|S|O
First it’s not that easy to read and second we do not validate the structure of options, any object is considered valid.
Such limitations made me to come up with an alternative - byContract. It is based on a similar principle, but instead of single-character signature it expects JSDoc expressions.
So the function from above can validated like that:
import { validate, typedef } from "bycontract";
function createWriteStream( path, options ) {
validate( arguments, ["string|Buffer", "string|WriteStreamOptions#" ]);
}
Meaning, we state that path can be a string or an instance of Buffer and options can be a string or an object of WriteStreamOptions type or missing (note # at the end of the signature). Here WriteStreamOptions is a custom type representing write stream options. We can define it as follows:
typedef( "WriteStreamOptions", {
flags: "string#",
encoding: "string#",
fd: "number#",
mode: "number#",
autoClose: "boolean#",
start: "number#"
});
I find it quite neat already, but can we do better? As a diligent developer you probably provide your functions with JSDoc. Why not to simply reuse it? Examine how you can do it with byContract:
import { validateJsdoc, typedef } from "bycontract";
class Fs {
@validateJsdoc(`
/**
* @param {string|Buffer} path
* @param {string|WriteStreamOptions} options
* @returns {<WriteStream>}
*/
`)
createWriteStream() {
}
}
With validate in byContract you can validate either list of arguments against a list of constraints or a single value against a single constraint. The function always returns incoming values(s). That can be used for example for exit point validation:
function pdf() {
//...
return validate( returnValue, "Promise" );
}
What if a function accepts different combinations of argument types? Let’s say we have compare function that takes in either two strings or two arrays of strings. We just need to use validateCombo:
import { validateCombo } from "bycontract";
function compare( a, b ) {
const CASE1 = [ "string", "string" ],
CASE1 = [ "string[]", "string[]" ];
validateCombo( arguments, [ CASE1, CASE2 ] );
}
But personally I like taking advantage of it when validting React.js action creators. Let’s say we define constraints in a separate module:
/interface/index.js
export const GROUP_REF = {
id: "string"
};
export const ENTITY = {
editing: "boolean=",
disabled: "boolean="
};
export const GROUP = {
title: "string=",
tests: "*[]",
...GROUP_REF
};
Now we can reuse them in actions:
import { validate } from "bycontract";
import * as I from "interface";
export const addGroup = ( data, ref = null ) => ({
type: constants.ADD_GROUP,
payload: {
data: validate( data, { ...I.ENTITY, ...I.GROUP } ),
ref: validate( ref, I.GROUP_REF )
}
});
As you see I combine abstract entity and group constraints { …I.ENTITY, …I.GROUP }. Thus when I need to validate another entity subclass I do for example { …I.ENTITY, …I.TEST } or { …I.ENTITY, …I.COMMAND }
Recap
byContract covers literally all of my needs for argument validation. It’s not that concise as aproba, but much more flexible and powerful.
One doesn’t need to learn a new constraint syntax given you know already JSDoc. It’s fast enough, but still can be turned off for production builds.
The library can be used as ES module, with Node.js and as none-module in a browser